Learn A-Z Computer Vision In 15 Days
In this lecture, we will about Image basics with Opencv.
Install OpenCV in python
!pip install opencv-python
-Read, Display & Write Image
Read the Image
import cv2
image = cv2.imread("Shape.png") # Give the image path
image = cv2.imread("Shape.png") # Give the image path
Display the Image
cv2.imshow("image_window",image)
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2.waitKey(0)
cv2.destroyAllWindows()
OR
import matplotlib.pyplot as plt
%matplotlib inline
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
%matplotlib inline
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
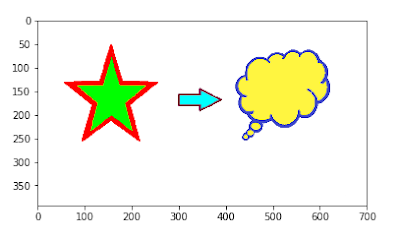
Read Grayscale(black-white) Image
import cv2
image = cv2.imread("Shape.png",0)
image = cv2.imread("Shape.png")
image = cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
image = cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
plt.imshow(image,cmap='gray')
Save the Image
cv2.imwrite('New_Grayscale_Picture.jpg',image)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(image,cmap='gray')
ax = fig.add_subplot(111)
ax.imshow(image,cmap='gray')
Draw Images
import cv2
import numpy as np
import numpy as np
cv2.imshow("black_bg",np.zeros(shape=(512,512,3),dtype=np.int8))
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2.waitKey(0)
cv2.destroyAllWindows()
OR
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(np.zeros(shape=(512,512,3),dtype=np.int8))
ax = fig.add_subplot(111)
ax.imshow(np.zeros(shape=(512,512,3),dtype=np.int8))
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(np.ones(shape=(512,512,3),dtype=np.int8)*255)
ax = fig.add_subplot(111)
ax.imshow(np.ones(shape=(512,512,3),dtype=np.int8)*255)
Draw the Line on Black Bg
# Draw a diagonal Green line of thickness of 5 pixels
image = np.zeros((450,750,3), np.uint8) # (height,width)
cv2.line(image, (0,0), (750, 450), (0,255,0), 5)
image = np.zeros((450,750,3), np.uint8) # (height,width)
cv2.line(image, (0,0), (750, 450), (0,255,0), 5)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
Draw Rectangle On Black Background
image = np.zeros((450,750,3), np.uint8) #(height,width)
cv2.rectangle(image,(150,100), (500, 300), (0,255,0), 5)
cv2.rectangle(image,(150,100), (500, 300), (0,255,0), 5)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
Draw Circle On Black Background
image = np.zeros((450,750,3), np.uint8) #(height,width)
cv2.circle(image,(350,200), 100, (0,255,0), 5)
cv2.circle(image,(350,200), 100, (0,255,0), 5)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
Draw Circle OnClick
import cv2
import numpy as np
# Create a function based on a CV2 Event
def draw_circle(event,x,y,flags,param):
if event == cv2.EVENT_LBUTTONDOWN: #(Left button click)
cv2.circle(img,(x,y),50,(0,255,0),5)
elif event == cv2.EVENT_RBUTTONDOWN: #(Right button click)
cv2.circle(img,(x,y),50,(0,0,255),5)
# Create a black image
img = np.zeros((450,750,3), np.uint8)
# This names the window so we can reference it
cv2.namedWindow(winname='my_drawing')
# Connects the mouse button to our callback function
cv2.setMouseCallback('my_drawing',draw_circle)
while True: #Runs forever until we break with Esc key on keyboard
# Shows the image window
cv2.imshow('my_drawing',img)
if cv2.waitKey(20) & 0xFF == 27:
break
# Once script is done, its usually good practice to call this line
# It closes all windows (just in case you have multiple windows called)
cv2.destroyAllWindows()
import numpy as np
# Create a function based on a CV2 Event
def draw_circle(event,x,y,flags,param):
if event == cv2.EVENT_LBUTTONDOWN: #(Left button click)
cv2.circle(img,(x,y),50,(0,255,0),5)
elif event == cv2.EVENT_RBUTTONDOWN: #(Right button click)
cv2.circle(img,(x,y),50,(0,0,255),5)
# Create a black image
img = np.zeros((450,750,3), np.uint8)
# This names the window so we can reference it
cv2.namedWindow(winname='my_drawing')
# Connects the mouse button to our callback function
cv2.setMouseCallback('my_drawing',draw_circle)
while True: #Runs forever until we break with Esc key on keyboard
# Shows the image window
cv2.imshow('my_drawing',img)
if cv2.waitKey(20) & 0xFF == 27:
break
# Once script is done, its usually good practice to call this line
# It closes all windows (just in case you have multiple windows called)
cv2.destroyAllWindows()
image = np.zeros((450,750,3), np.uint8)
cv2.putText(image, 'Hello Mayank!', (200,200), cv2.FONT_HERSHEY_SIMPLEX, 2, (0,255,0), 3)
cv2.putText(image, 'Hello Mayank!', (200,200), cv2.FONT_HERSHEY_SIMPLEX, 2, (0,255,0), 3)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111) ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
Color Space
- BGR format generates the color by combining Blue, Green & Red. Opencv stores color in BRG.
- RGR format generates the color by combining Blue, Green & Red. PIL stores color in BRG.
a = 1
import cv2 import matplotlib.pyplot as plt import numpy as np %matplotlib inline
# Read image in BGR format
image = cv2.imread("target.jpg")
cv2.imshow("image_window",image)
cv2.waitKey(0)
cv2.destroyAllWindows()
image = cv2.imread("target.jpg")
cv2.imshow("image_window",image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# Convert the BGR to RGB
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow()
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow()
# Read image in BGR format
image = cv2.imread("target.jpg")
B, G, R = cv2.split(image)
zeros = np.zeros(image.shape[:2], dtype = "uint8")
cv2.imshow("Blue", cv2.merge([B, zeros, zeros]))
cv2.imshow("Green", cv2.merge([zeros, G, zeros]))
cv2.imshow("Red", cv2.merge([zeros, zeros, R]))
cv2.waitKey(0)
cv2.destroyAllWindows()
image = cv2.imread("target.jpg")
B, G, R = cv2.split(image)
zeros = np.zeros(image.shape[:2], dtype = "uint8")
cv2.imshow("Blue", cv2.merge([B, zeros, zeros]))
cv2.imshow("Green", cv2.merge([zeros, G, zeros]))
cv2.imshow("Red", cv2.merge([zeros, zeros, R]))
cv2.waitKey(0)
cv2.destroyAllWindows()
fig,axes = plt.subplots(nrows= 1, ncols = 3,dpi=300 )
axes[0].imshow(cv2.cvtColor(cv2.merge([B, zeros, zeros]), cv2.COLOR_BGR2RGB))
axes[1].imshow(cv2.cvtColor( cv2.merge([zeros, G, zeros]), cv2.COLOR_BGR2RGB))
axes[2].imshow(cv2.cvtColor( cv2.merge([zeros, zeros, R]), cv2.COLOR_BGR2RGB))
for ax in axes:
ax.set_xticks([])
ax.set_yticks([])
axes[0].imshow(cv2.cvtColor(cv2.merge([B, zeros, zeros]), cv2.COLOR_BGR2RGB))
axes[1].imshow(cv2.cvtColor( cv2.merge([zeros, G, zeros]), cv2.COLOR_BGR2RGB))
axes[2].imshow(cv2.cvtColor( cv2.merge([zeros, zeros, R]), cv2.COLOR_BGR2RGB))
for ax in axes:
ax.set_xticks([])
ax.set_yticks([])
# Read image in BGR format
image = cv2.imread("target.jpg")
image[:,:,0] = 0 # Zero out contribution from blue
image[:,:,1] = 1 # Zero out contribution from green
image = cv2.imread("target.jpg")
image[:,:,0] = 0 # Zero out contribution from blue
image[:,:,1] = 1 # Zero out contribution from green
# Matplot reuires RGB format
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111)
ax.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
-HSV format closely aligns with the way human vision perceives color-making attributes. It has:
- Hue - color value(0-179)
- Saturation- Gray color intensity (0-255)
- Value - Brightness (0-255)
Color filtering Using HSV
import cv2
import numpy as np
image = cv2.imread('target.jpg')
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
# Look the Color to Define the Range the Blue Color
# lower range for blue color is 95. So the lower limit for HSV is (95 ,0 ,0)
# Upper range for blue color is 95. So the lower limit for HSV is (120 ,255 ,255)
mask = cv2.inRange(hsv, (95,0,0), (120,255,255))
import numpy as np
image = cv2.imread('target.jpg')
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
# Look the Color to Define the Range the Blue Color
# lower range for blue color is 95. So the lower limit for HSV is (95 ,0 ,0)
# Upper range for blue color is 95. So the lower limit for HSV is (120 ,255 ,255)
mask = cv2.inRange(hsv, (95,0,0), (120,255,255))
fig,axes = plt.subplots(nrows= 1 , ncols = 2,dpi=200 )
axes[0].imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
axes[1].imshow(cv2.cvtColor(mask, cv2.COLOR_BGR2RGB))
axes[0].imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
axes[1].imshow(cv2.cvtColor(mask, cv2.COLOR_BGR2RGB))
![]() |
Original Image Segment Image for Blue |
In the next blog, we will discuss the Read, Show & Write Image.
https://sngurukuls247.blogspot.com/2020/05/computer-vision-3-image-basics-with.html
.
https://www.youtube.com/channel/UCENc9qI7_r8KMf6-_1R1xnw
https://sngurukuls247.blogspot.com/2020/05/computer-vision-3-image-basics-with.html
.
Follow the link below to access Free Python Lectures-
https://www.youtube.com/channel/UCENc9qI7_r8KMf6-_1R1xnw
Instagram-
No comments:
Post a Comment